1)Introduction
A programmer can control code flow in a C code using several techniques. Before start coding one should have an idea about the code flow for that particular code. Code flow is purely the logic required for the program. The programmer converts the logic into C code, and he may use the code flow control techniques available in the C during the process.
I hope this tutorial will give you a solid idea about the code flow control. The examples actually shows how can you make use of the conditions, loops etc.
2)Â Â Conditional branching
In conditional branching, a decision is made to direct the code flow through any one of code blocks based on a condition check
2.1)Â Â If else
If else syntax:
If ( condition )
{
Code block for condition TRUE
}
else
{
Code block for condition FALSE
}
If else code flow:
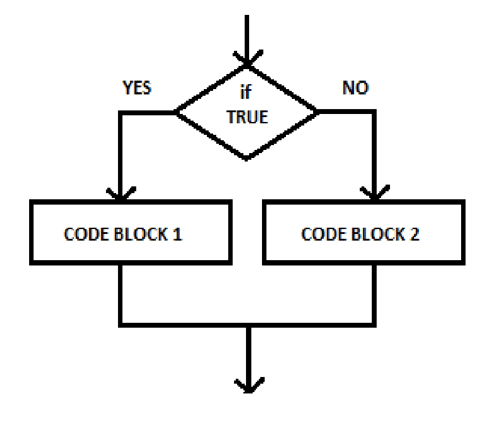
[sam id=”5″ codes=”true”]
If the condition is true code block 1 will get executed, otherwise code block 2 will get executed.
If else examples:
C_2.1.1 :Â Simple if else
int main ()
{
char *name = “sachin”;
if ( name == “sachin” )
write ( 0, “\ngreat cricketer\n”, 17 );
else
write ( 0, “\ndont know\n”, 11 );
}
Output:
great cricketer
C_2.1.2 : Else block not necessary
int main ()
{
char *name = “sachin”;
if ( name == “sachin” )
write ( 0, “\ngreat cricketer\n”, 17 ); else;
}
Output:
great cricketer
C_2.1.3 : Nested if else
#include <stdio.h>
int main ()
{
char *name = “Emma Watson”;
if ( name == “sachin” )
{
printf ( “\ngreat cricketer\n” ); printf ( ” true indian\n” );
}
else if ( name == “Jackie Chan” )
{
printf ( “\ngreat actor” );
printf ( ” great fight master\n” );
}
else if ( name == “A R Rehman” ) printf ( “\ngreat musician\n” );
else if ( name == “Emma Watson” ) printf ( “\nHarry Porter Girl\n” );
else
printf ( “\nI dont know who\n” );
}
Output:
Harry Porter Girl
2.2) Using conditional operator
The code flow can be controlled by using the conditional operator also.
Syntax for using conditional operator:
( condition ) ? ( code block for condition TRUE ) : ( code block for condition FALSE )
Conditional operator examples :
C_2.2.1 : Code equivalent to C_2.1.1
int main ()
{
char *name = “sachin”;
( name == “sachin” ) ? write ( 0, “\ngreat cricketer\n”, 17 ) : write ( 0, “\ndont know\n”, 11 );
}